【FastAPI】パラメータ・リクエストボディの使い方【入門】
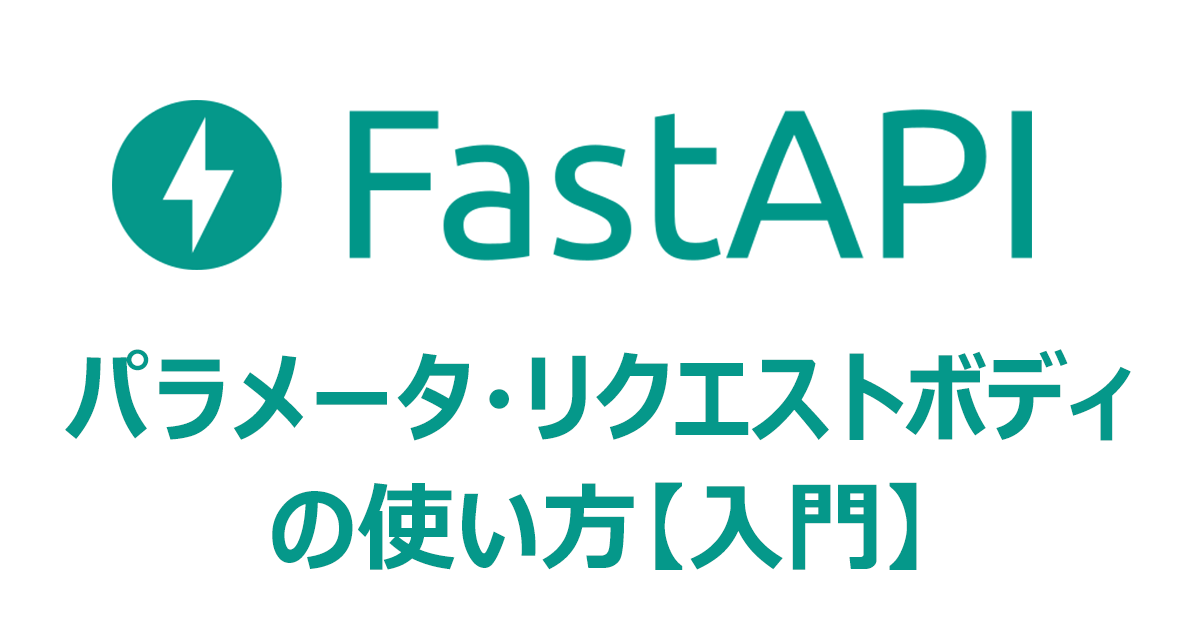
FastAPI
では、簡単に受け取るパラメーターやリクエストボディの記述が可能です。
今回は、パス・クエリパラメーターやリクエストボディの記述方法をご紹介いたします。
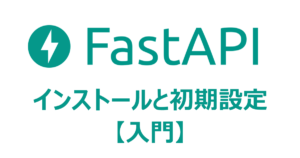
FastAPIの基礎についての記事まとめ
パスパラメーターの付与
URL
に任意のパラメーターを受け取る場合には、{id}
と引数を受け取ります。
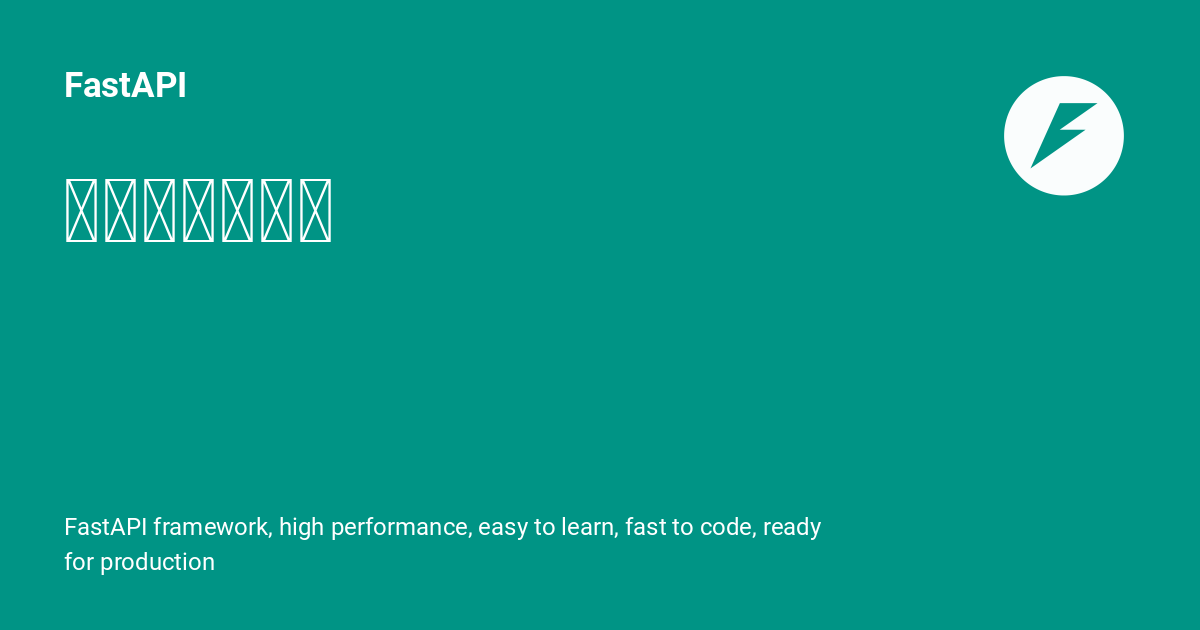
@app.get('/article/{id}')
def get_article(id):
return {'message': f'article is {id}'}
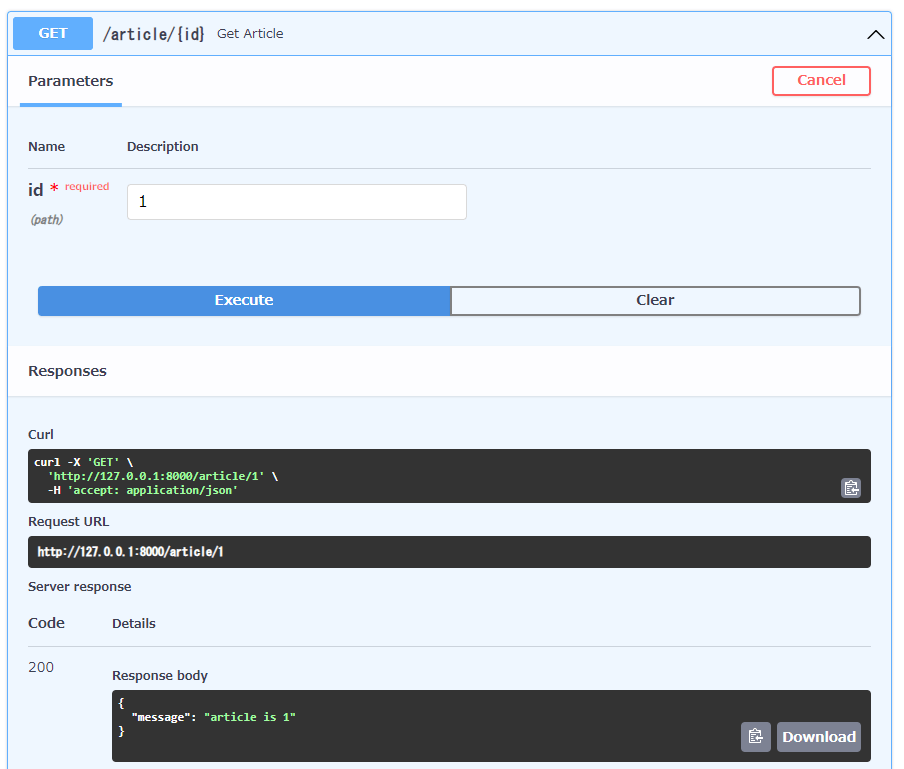
受け取るパラメーターは、int, str, bool
などで型指定が可能です。
@app.get('/article/{id}')
def get_article(id: int): <-- int
return {'message': f'article is {id}'}
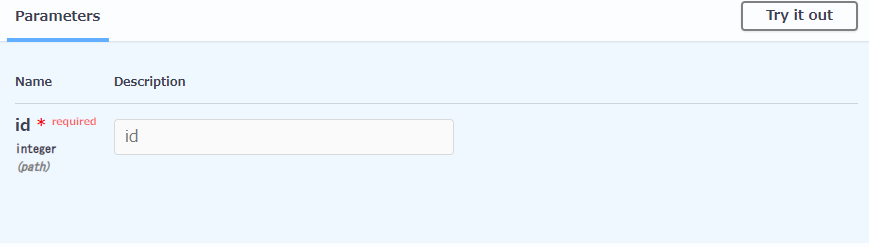
複数のパラメータを受け取ることも可能です。
@app.get('/article/{id}/comments/{comment_id}')
def get_article(id: int, comment_id: int):
return {'message': f'article is {id} and {comment_id}'}
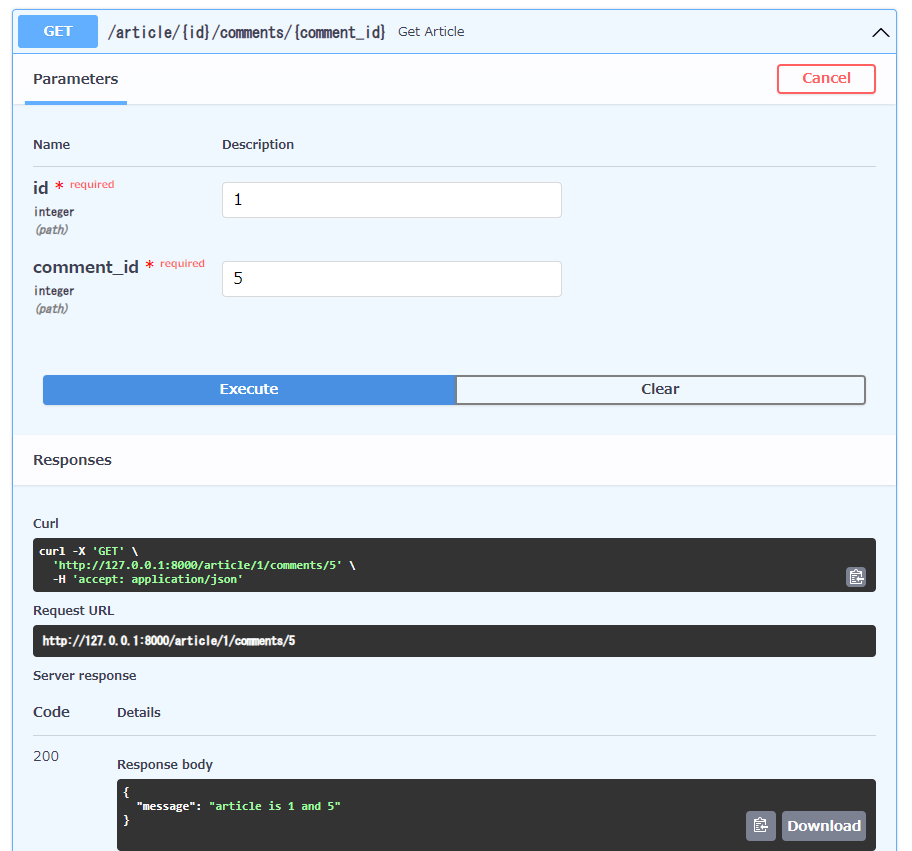
クエリパラメーターの付与
http://127.0.0.1/article/all?username=name&is_display=true
のようなクエリパラメーターを付与する場合も同様です。
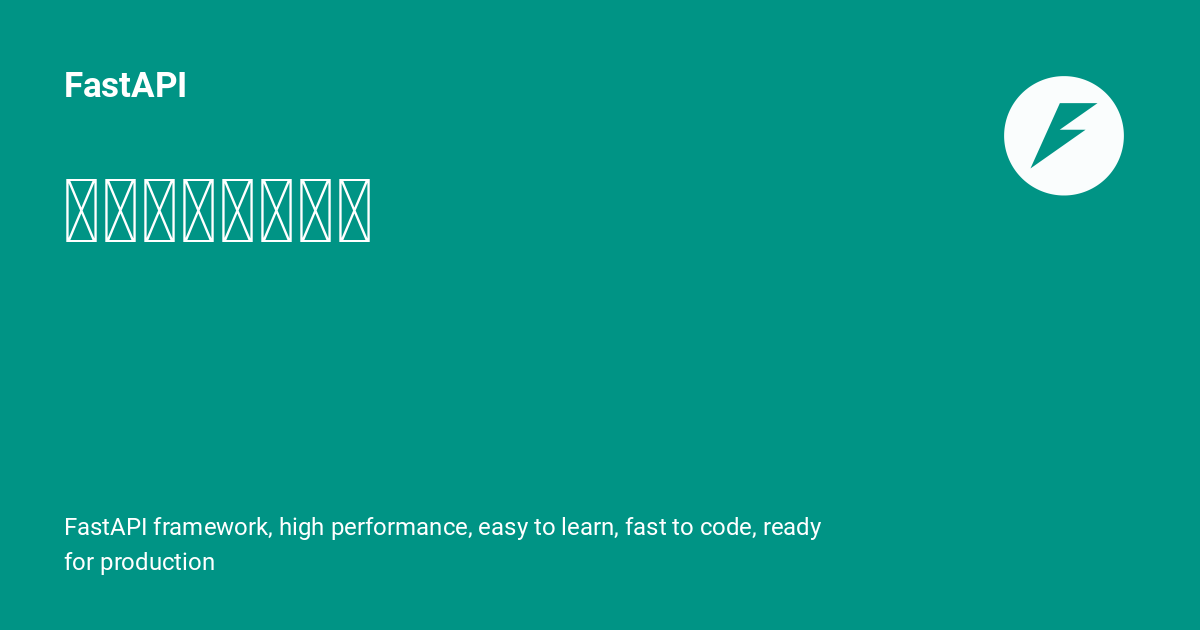
@app.get('/article/all')
def get_article(username: str, is_display: bool = True):
return {'message': f'article all query {username} and {is_display}'}
リクエストボディ
POST
メソッドなどでボディを受け取る場合には、pydantic
からBaseModel
をインポートして継承したクラスを作成します。
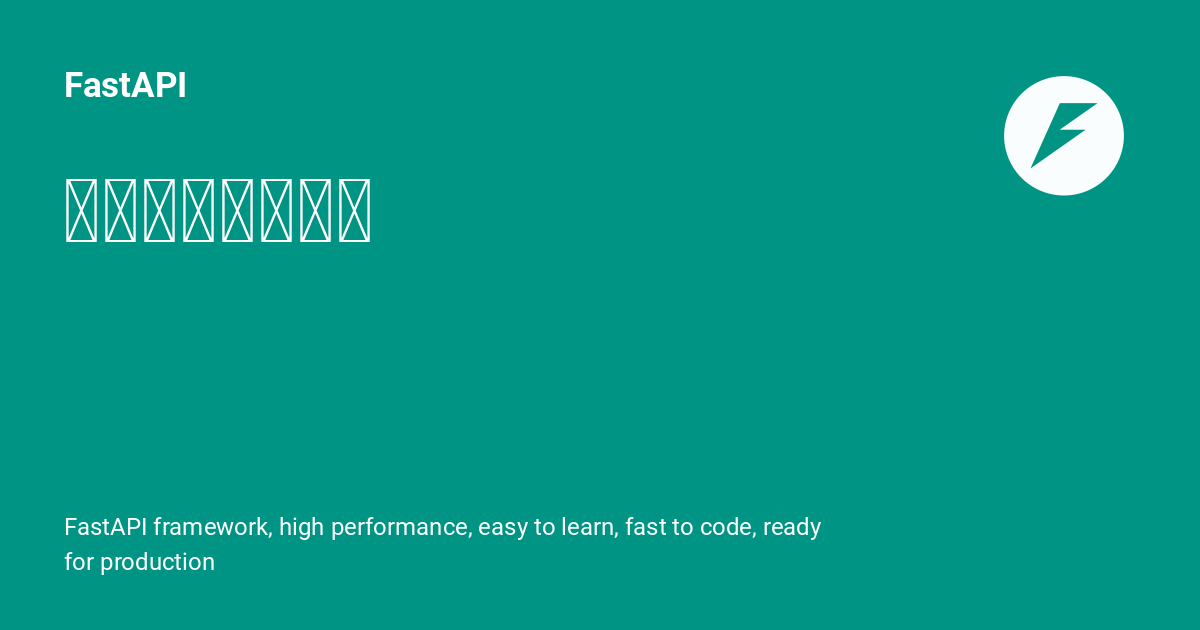
作成したモデルを、aritcle: ArticleModel
としています。
from pydantic import BaseModel
from typing import Optional
~~~
class ArticleModel(BaseModel):
title: str
content: str
username: str
is_display: Optional[bool]
@app.post('/article')
def get_article(article: ArticleModel, username: Optional[str] = None, is_display: bool = True):
return {
'article': article,
'username': username,
'is_display': is_display
}
ドキュメントを起動して、試しに送信してみましょう。適当な文字列を入力しました。
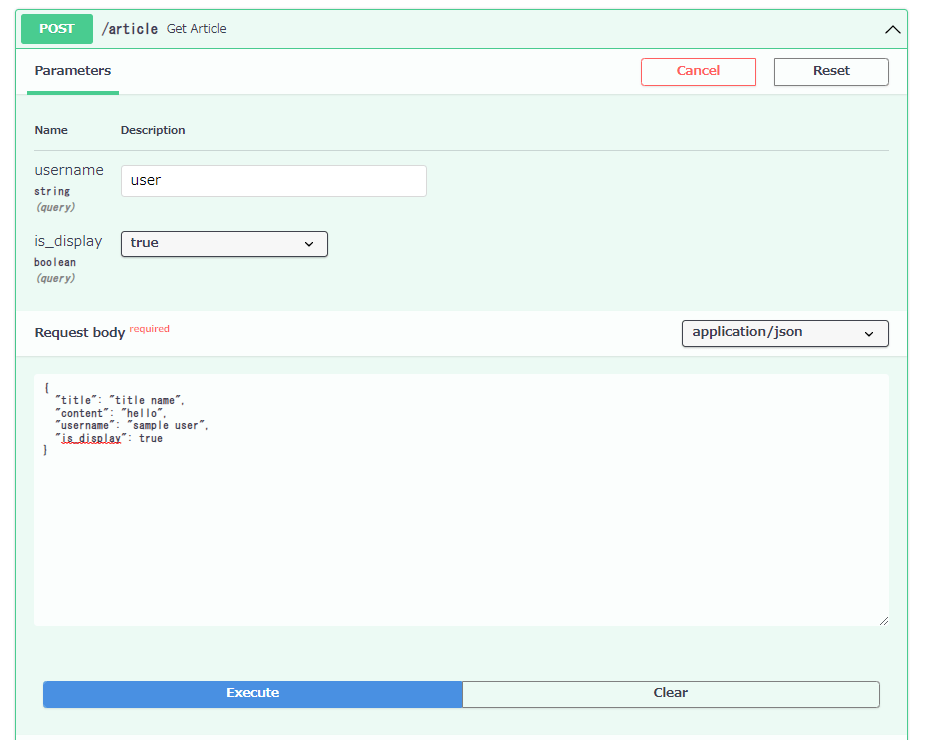
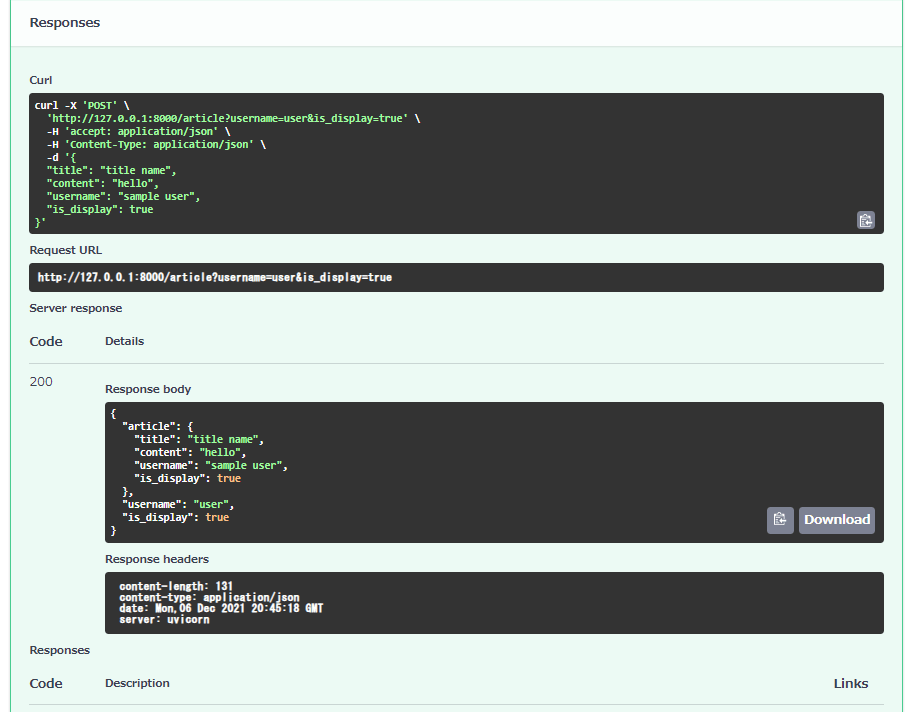
パス・クエリパラメーター、リクエストボディ全てを受け取れました。
複雑なクエリパラメーターやリクエストボディの記述方法
必須にしない(Optional)
クエリパラメーターやリクエストボディを必須にしない場合には、python
の型ヒントを活用してOptional
を用います。
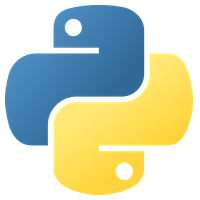
今回の例ではusername
をusername: Optional[str] = None
とし、任意の値に指定します。
from typing import Optional
~~~
@app.get('/article/all')
def get_article(username: Optional[str] = None, is_display: bool = True):
return {'message': f'article all query {username} and {is_display}'}
ドキュメントを確認すると必須ではなくなっていることが確認できました。
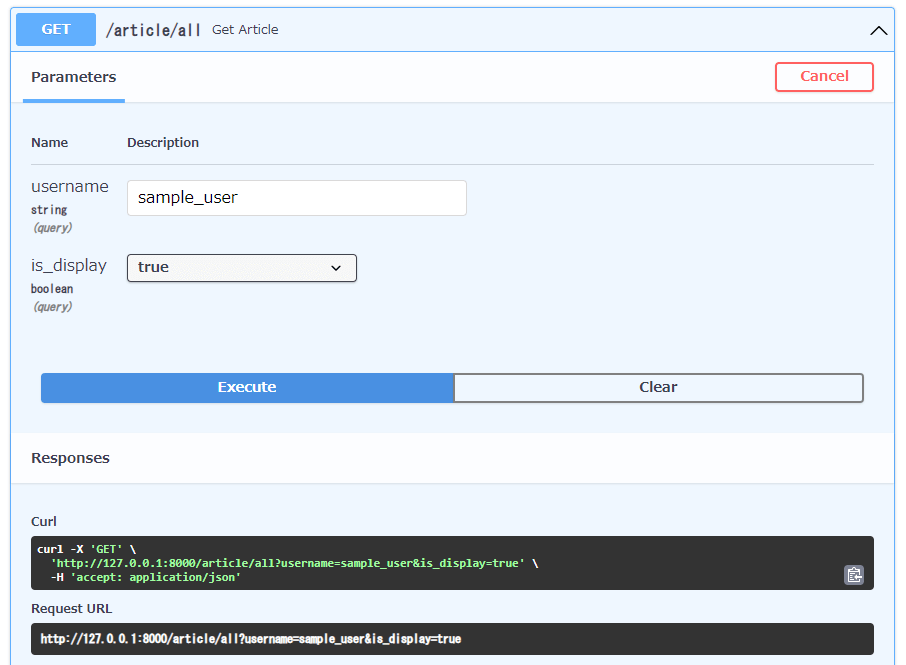
リストや辞書形式での定義(List, Dict)
リストや辞書形式など複雑な構造を持つ場合には、typing
からList
やDict
をインポートします。
from pydantic import BaseModel
from typing import Optional, List, Dict
~~~
class ArticleModel(BaseModel):
title: str
content: str
username: str
tag: List[str] = []
metadata: Dict[str, str] = {'key': 'value'}
@app.post('/article')
def get_article(article: List[ArticleModel], username: Optional[str] = None, is_display: bool = True):
return {
'article': article,
'username': username,
'is_display': is_display
}
ドキュメントを確認すると、リスト、辞書型が受け取れました。
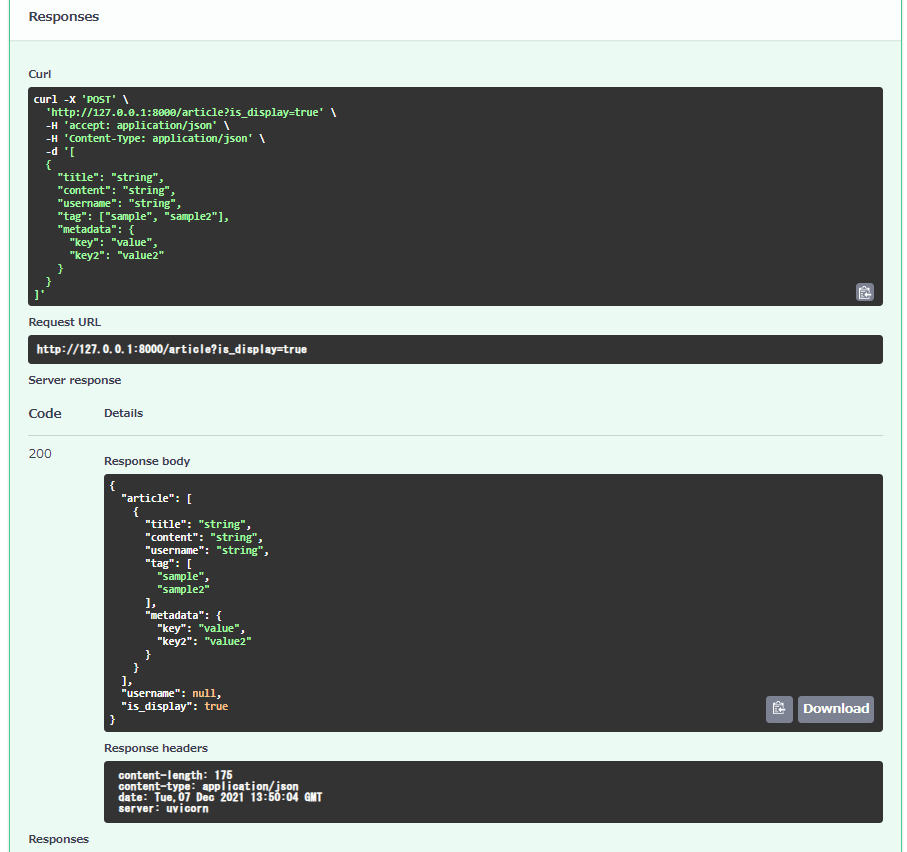
metaデータやバリデーションの追加
クエリパラメータを明示して、様々なオプションを活用する場合にはfastapi
から
Path
パスパラメーターQuery
クエリパラメーターBody
リクエストボディ
をそれぞれインポートします。引数に関してはほとんど共通です。
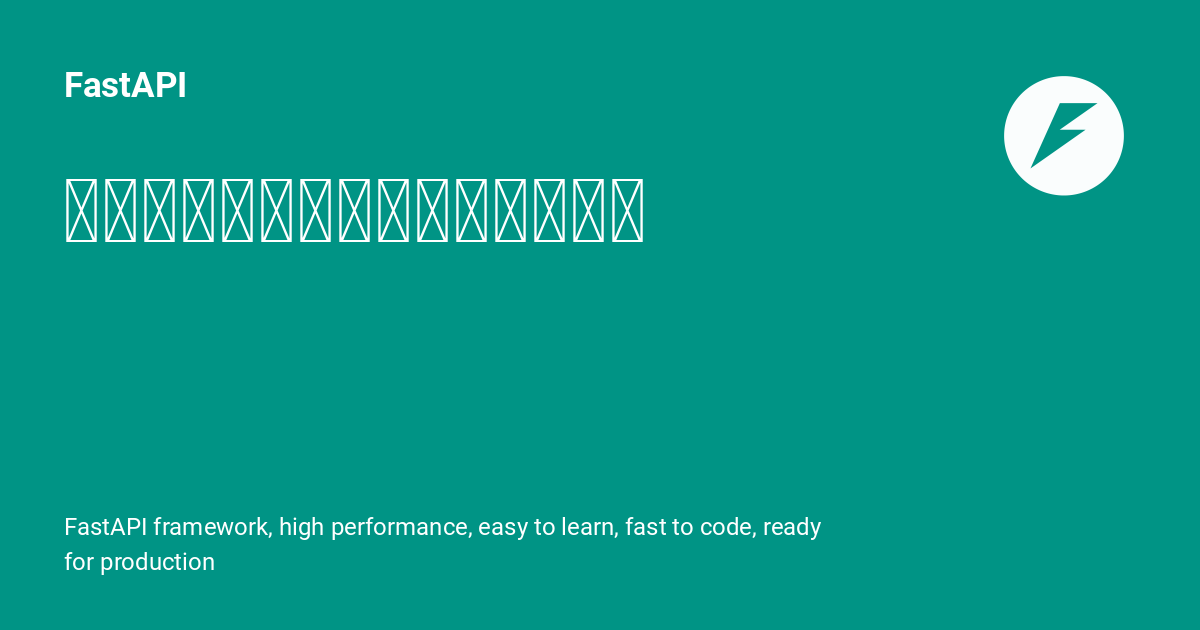
これらを活用すると
- 複数のリストを値を受け取る
- metaデータの追加
alias
title
description
deprecated
- 文字列のバリデーション
min_length
max_length
regex
- 数値のバリデーション
gt
:g
reatert
hange
:g
reater than ore
quallt
:l
esst
hanle
:l
ess than ore
qual
メタデータやバリデーションの追加が可能です。
FastAPIの基礎についての記事まとめ
コメント