【FastAPI】エラー処理の追加【入門】
当ページのリンクには広告が含まれています。
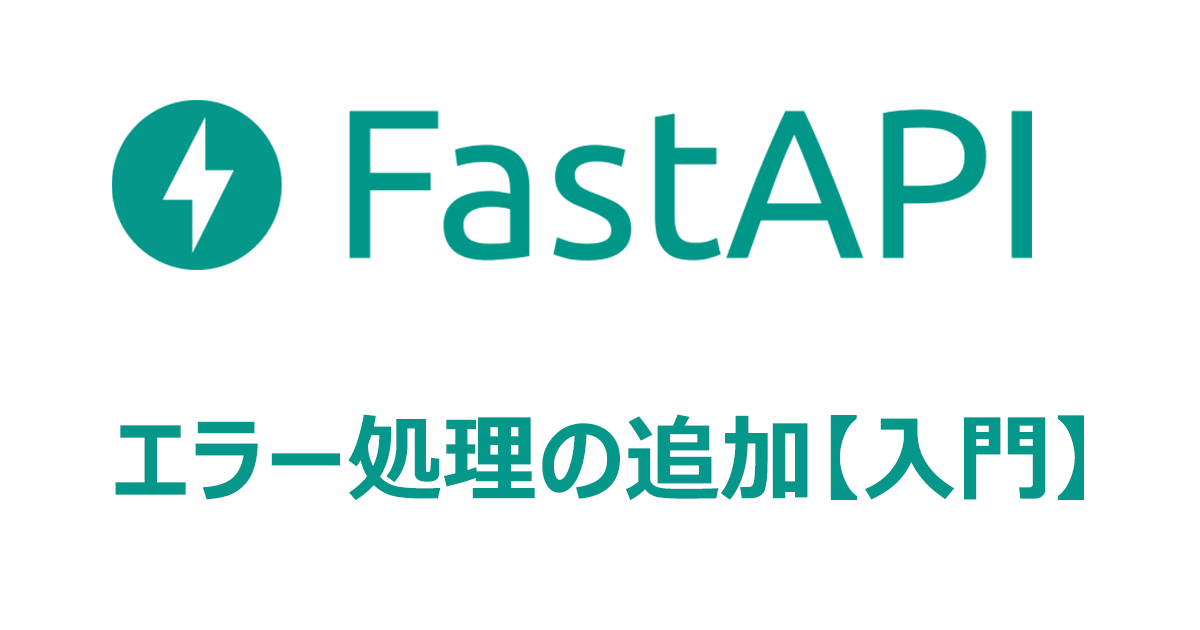
APIを作成する際に、クライアント側にエラーを伝えることはとても重要なことです。
例えば
- クライアントに権限が無い事
- データベースに値が無い事
等があります。
今回は、例外処理の追加やステータスコードの付与についてご紹介いたします。
あわせて読みたい

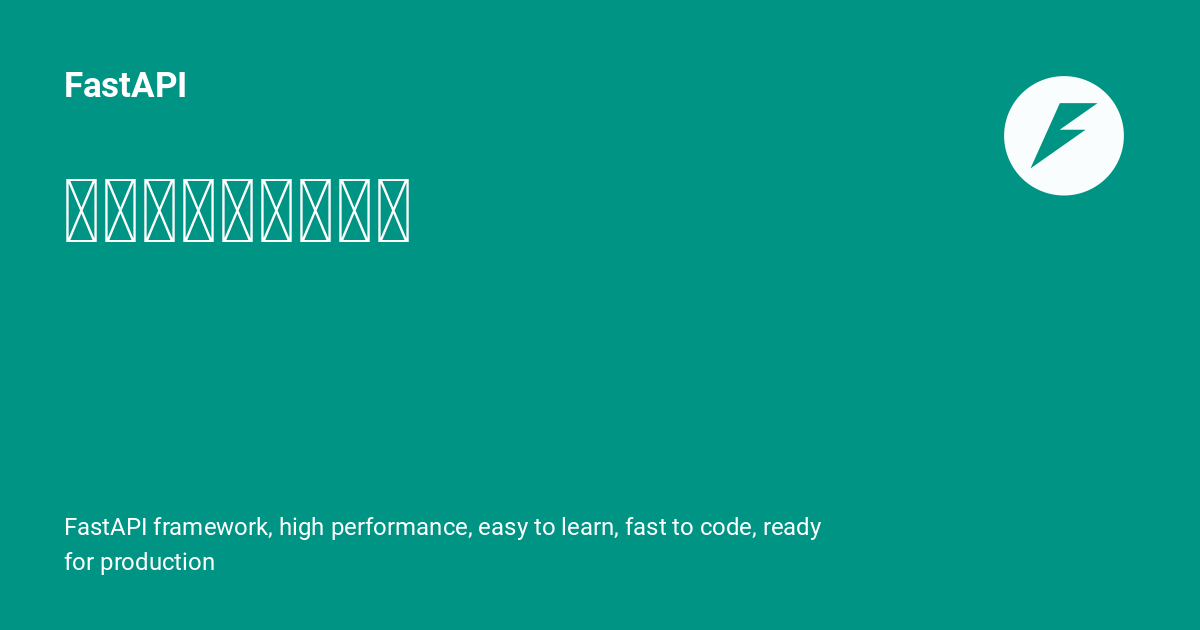
エラーハンドリング – FastAPI
FastAPI framework, high performance, easy to learn, fast to code, ready for production
関連記事

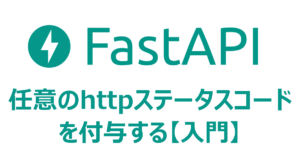
【FastAPI】任意のhttpステータスコードを付与する【入門】
代表的なステータスコードには 成功(Success)の200未検出(Not Found)の404サーバーエラー(Internal Server Error)の500 等がありますが、レスポンスがどのような状態な…
FastAPIの基礎についての記事まとめ
目次
基本形
サンプルコードとして、Article
のモデルを作成し、新規作成・1件取得のAPIを作成しました。
from sqlalchemy.orm.session import Session
from schemas import ArticleBase
from db.models import Article
def create_article(db: Session, request: ArticleBase):
new_article = Article(
title = request.title,
content = request.content,
is_display = request.is_display,
creater_id = request.creater_id
)
db.add(new_article)
db.commit()
db.refresh(new_article)
return new_article
def get_article(db: Session, id: int):
article = db.query(Article).filter(Article.id == id).first()
return article
新規作成と、任意のID
の記事を取得できるものです。router
は下記になります。
from fastapi import APIRouter, Depends
from sqlalchemy.orm import Session
from db.database import get_db
from db import db_article
from schemas import ArticleBase, ArticleDisplay
router = APIRouter(
prefix='/article',
tags=['article']
)
@router.post('/', response_model=ArticleDisplay)
def create_article(request: ArticleBase, db: Session = Depends(get_db)):
return db_article.create_article(db, request)
@router.get('/{id}', response_model=ArticleDisplay)
def get_article(id: int, db: Session = Depends(get_db)):
return db_article.get_article(db, id)
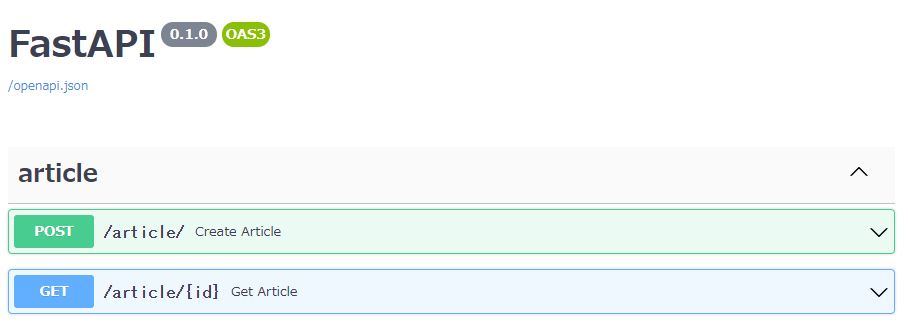
例外処理の追加 – HttpException
データベースに値が無かった場合に404エラー
記事を参照する/article/{id}
で指定したID
に記事が無かった場合はどうなるのでしょうか?。
現在は、記事があった場合にはステータスコード200
番が返され記事情報がレスポンスになりますが、記事が無い場合にはnull
が返ります。
今回は、null
ではなく、404
のステータスコードと任意のエラーメッセージを返すようにします。
from fastapi import HTTPException, status
~~~
@router.get('/{id}', response_model=ArticleDisplay)
def get_article(id: int, db: Session = Depends(get_db)):
article = db_article.get_article(db, id)
if not article:
raise HTTPException(
status_code=status.HTTP_404_NOT_FOUND,
detail=f"Article with id {id} not found"
)
return article
router/articles.py
のget
メソッドに処理を追加しました。
raise HTTPException(status_code=status.HTTP_404_NOT_FOUND, detail=f"Article with id {id} not found")
fastapi
からHTTPException
とstatus
をインポートして、ステータスコードとメッセージを記載しています。
fastapi
でのステータスコードについては下記記事をご参照ください。
関連記事

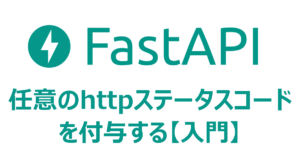
【FastAPI】任意のhttpステータスコードを付与する【入門】
代表的なステータスコードには 成功(Success)の200未検出(Not Found)の404サーバーエラー(Internal Server Error)の500 等がありますが、レスポンスがどのような状態な…
実装確認
ドキュメントで成功時と失敗時のレスポンスを確認してみましょう。
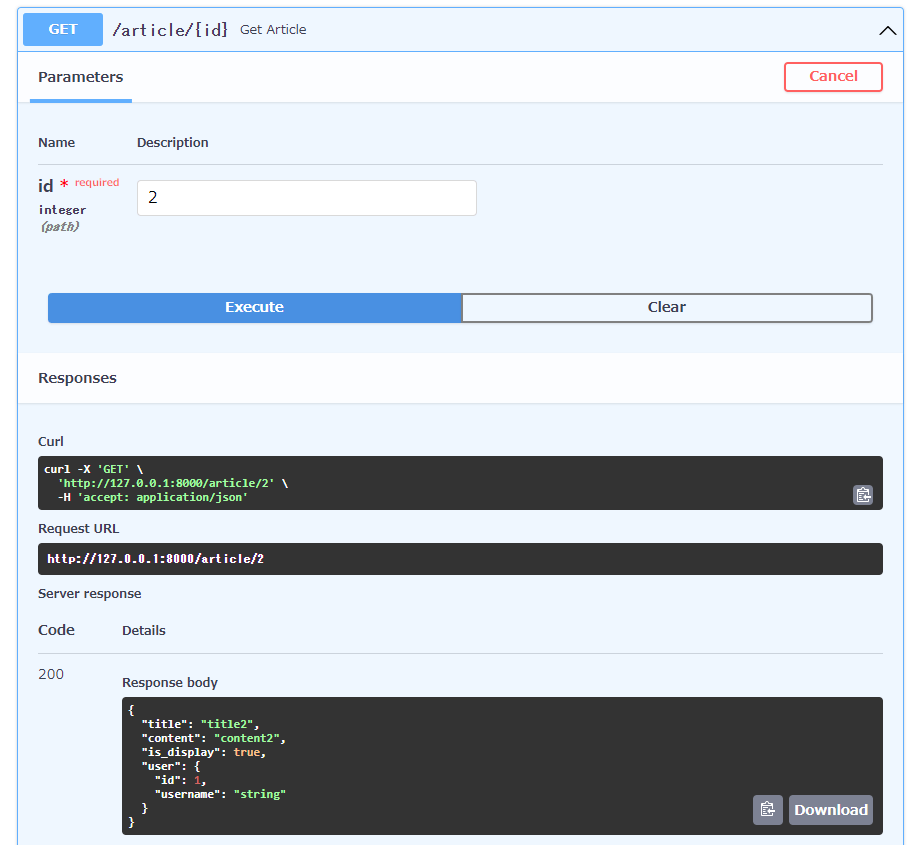
ID
が2
の時には、ステータスコードは200
番でレスポンスのデータも返ってきています。
{
"title": "title2",
"content": "content2",
"is_display": true,
"user": {
"id": 1,
"username": "string"
}
}
次にデータベースに存在しないID
での失敗時を確認してみましょう。
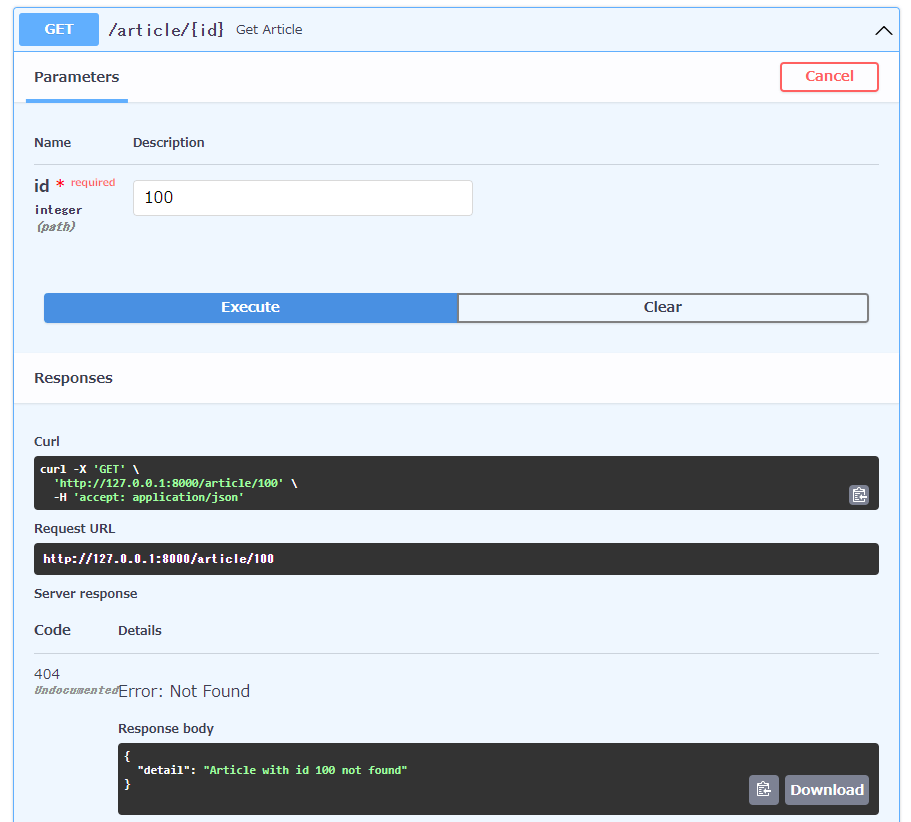
ステータスコードは404
番、メッセージも先ほど作成したものになっていますね!
{
"detail": "Article with id 100 not found"
}
FastAPIの基礎についての記事まとめ
コメント